Streamline API Testing with RemixPapa MSW
RemixPapa MSW is a versatile and efficient API mocking tool designed for modern web developers. Built on the robust Mock Service Worker (MSW) library, it enables developers to intercept HTTP requests and simulate API responses. Whether you’re mocking RESTful APIs, GraphQL endpoints, or handling client-side request behaviors, this tool simplifies the development and testing process.
When building web applications, relying on live APIs can introduce delays, errors, or interruptions. By simulating API responses with RemixPapa MSW, developers can work seamlessly without depending on backend services. This makes it an essential tool for frontend development, particularly for those using frameworks like Remix.
RemixPapa MSW stands out because of its smooth integration with the Remix framework and its focus on realistic API response simulation. Developers can test network requests, debug efficiently, and even simulate complex HTTP request scenarios. In this guide, we’ll explore the features, setup, and best practices for using RemixPapa MSW to optimize web application testing.
Aspect | Fact | Figure |
---|
Tool Name | RemixPapa MSW | – |
Core Functionality | API mocking for HTTP requests (GET, POST, PUT, DELETE), simulating API responses | – |
Supported API Types | RESTful APIs, GraphQL | – |
Integration | Seamless with Remix framework; also works with React, Jest, Cypress | – |
Error Simulation | Supports simulating API errors (e.g., 404, 500) | Example: Simulating 404 error for /api/users endpoint |
Mock Handler Types | GET, POST, PUT, DELETE | Example: Mocking GET request for /api/users |
Service Worker Setup | Requires setupWorker from MSW, initialized in the main entry file | worker.start() to activate service worker |
Simulating Complex Scenarios | Supports mocking dynamic responses based on parameters or conditions | – |
Debugging Tools | Built-in logging for intercepted HTTP requests and responses | – |
Testing Edge Cases | Allows simulation of different error scenarios like 404 and 500 responses | Example: Simulating a 500 error for /api/users |
Resource Library | Includes pre-built templates, sound effects, and visual components for rapid development | – |
Use in Staging Environments | Can be deployed in staging to test features without live APIs | – |
Installation | Install with npm install msw | – |
Service Worker for Production | Must be disabled in production to avoid conflicts with live APIs | – |
Supported Frameworks | Works with frontend frameworks like React, Remix | – |
Testing Without Backend | Allows testing and debugging without the need for live backends | – |
Monitoring Requests | Provides tools to monitor intercepted HTTP requests and view detailed logs | – |
Tool Flexibility | Adapts to small projects or enterprise-level applications | – |
Mocking Advanced Scenarios | Supports mocking API responses with complex conditions, like dynamic JSON data | – |
Frontend Developer Benefit | Enables frontend developers to continue working even if the backend is unavailable, speeding up development | – |
Production Use | Not recommended for production environments | – |
Features and Capabilities of the Platform
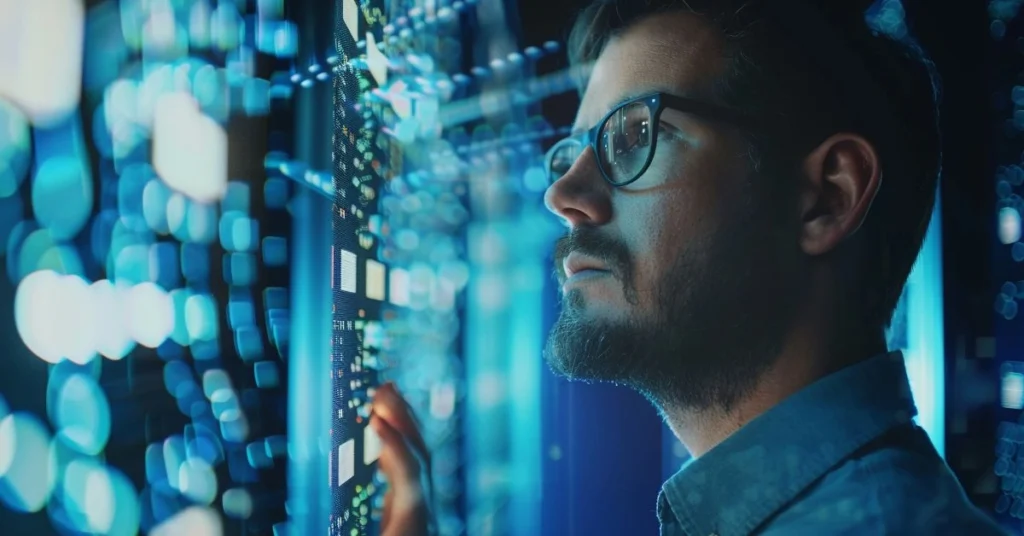
HTTP Request Interception and Handlers
One of the key features of this API testing tool is its ability to handle a wide range of HTTP methods, including GET, POST, PUT, and DELETE. With its service worker API, RemixPapa MSW enables developers to define detailed handlers for every type of request. These handlers allow the simulation of real-world scenarios, from fetching data to submitting forms, ensuring web application testing is as thorough as possible.
For example, developers can mock GraphQL APIs or RESTful APIs with ease. Handlers are flexible and can return specific responses based on parameters or conditions, helping simulate various network behaviors.
Seamless Integration with the Remix Framework
RemixPapa MSW integrates effortlessly with the Remix framework, aligning perfectly with its data-fetching model. This makes it an ideal choice for testing API calls in Remix applications. Developers using this tool benefit from seamless API mocking while maintaining a clean project structure.
Moreover, it works well alongside other popular frontend development tools like React, Jest, or Cypress, providing a comprehensive testing setup. Whether you’re building small-scale projects or enterprise-level applications, RemixPapa MSW adapts to your workflow.
Extensive Resource Library
The platform also offers an extensive resource library that includes pre-built templates, sound effects, and visual components. These resources make it easier for developers to enhance their projects quickly, especially when working on dynamic or interactive features.
This library reduces the need for building assets from scratch, saving valuable time in development. It’s particularly helpful for teams aiming to deliver high-quality web applications under tight deadlines.
Debugging and Testing Features
Debugging network requests is simplified with RemixPapa MSW’s built-in tools. Developers can monitor intercepted HTTP requests and view detailed logs of mocked responses. These tools provide insights into client-side request handling and help identify potential issues in real time.
Additionally, the platform supports advanced testing scenarios, such as simulating API errors. By mocking API errors like 404 or 500, developers can ensure their applications handle edge cases gracefully. This enhances the reliability of web application testing.
Setting Up and Using the Tool
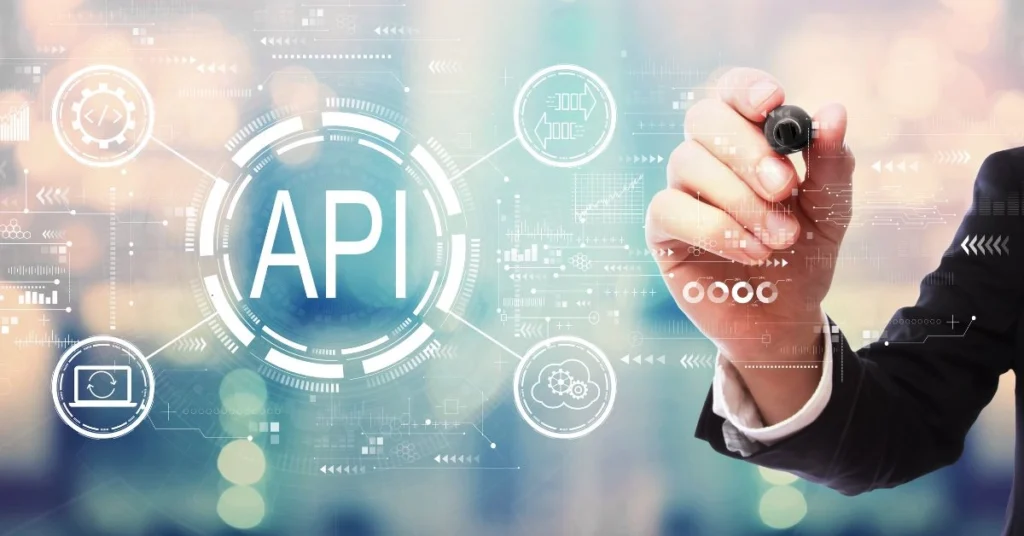
Installation and Configuration
To start using this API mocking tool, install it with the following command:
npm install msw
Next, create a src/mocks folder in your project to store handlers. Define mock API handlers in a file like handlers.js. For example, to simulate a successful GET request, you can write:
javascript
import { rest } from ‘msw’;
export const handlers = [
rest.get(‘/api/users’, (req, res, ctx) => {
return res(
ctx.status(200),
ctx.json([{ id: 1, name: ‘John Doe’ }])
);
}),
];
This mock response is perfect for testing network requests in development environments.
Starting the Service Worker
Once your handlers are ready, initialize the service worker in your main entry file:
javascript
import { setupWorker } from ‘msw’;
import { handlers } from ‘./mocks/handlers’;
const worker = setupWorker(…handlers);
worker.start();
This step ensures that the service worker API is active in your development environment. Remember to disable it in production to avoid conflicts with live APIs.
Simulating Errors and Testing Edge Cases
RemixPapa MSW makes it easy to simulate error states for API testing. For example, you can simulate a 404 response like this:
javascript
rest.get(‘/api/users’, (req, res, ctx) => {
return res(ctx.status(404), ctx.json({ error: ‘Not Found’ }));
});
This allows you to test how your application handles failed API calls, ensuring a better user experience.
Using Mock APIs in Staging
Mock APIs are not just useful during development. They can also be deployed in staging environments to test features without relying on live APIs. This ensures that your testing process remains isolated from external factors, improving the reliability of your tests.
Conclusion
API mocking tools are indispensable for modern web development, streamlining the process of building, testing, and debugging applications. By intercepting HTTP requests and simulating realistic API responses, these tools allow developers to work independently of live backends, reducing delays and improving efficiency. With features like HTTP request handling, seamless framework integration, and error simulation, such tools are particularly valuable for testing edge cases and ensuring application reliability.
Their flexibility and ease of use make them essential for teams looking to enhance productivity and deliver high-quality projects. Whether you’re simulating RESTful APIs or GraphQL endpoints, these solutions provide a robust foundation for creating and testing web applications in any development environment.
What is an API mocking tool, and why is it useful?
An API mocking tool intercepts HTTP requests and simulates responses, allowing developers to test and debug applications without relying on live backends. It’s useful for ensuring smooth development, testing edge cases, and avoiding delays caused by backend dependencies.
How does this tool handle HTTP requests?
The tool uses handlers to intercept HTTP methods like GET, POST, PUT, and DELETE. Developers can define specific responses for each request, simulating real-world scenarios and customizing behavior based on parameters or conditions.
Can this tool integrate with frontend frameworks like React?
Yes, it integrates seamlessly with popular frameworks like React. It also works well alongside tools like Jest and Cypress, providing a comprehensive environment for testing web applications.
How do I set up this tool in my project?
First, install the necessary library using your package manager. Then, create a folder for mock handlers and define responses for specific endpoints. Finally, initialize the service worker in your entry file to start intercepting requests.
Can this tool simulate API errors?
Yes, you can easily simulate API errors like 404 (Not Found) or 500 (Internal Server Error). This allows developers to test how their applications handle failed requests and improve user experience during error scenarios.
Is it safe to use this tool in production?
No, API mocking tools are meant for development and testing environments. Ensure the service worker is disabled in production to avoid conflicts with live APIs.
What are the advantages of using mock APIs in staging environments?
Mock APIs in staging environments allow for isolated testing, ensuring features work as intended without relying on external backend services. This improves reliability and helps identify issues before deploying to production.
How can I monitor intercepted HTTP requests?
The tool provides built-in debugging capabilities, allowing developers to view detailed logs of intercepted requests and responses. This makes it easier to identify issues and verify application behavior.
Does this tool support GraphQL APIs?
Yes, it supports both RESTful APIs and GraphQL endpoints. Developers can define handlers specifically for GraphQL queries and mutations to test data-fetching scenarios effectively.
Why should I use an API mocking tool instead of live APIs during development?
Live APIs can introduce delays, errors, or downtime, disrupting development workflows. Mock APIs ensure that your work isn’t dependent on backend availability, providing a stable, controlled environment for building and testing applications.